How to Filter Object Properties in JavaScript
A simple JavaScript polyfill of the filter array method applied to object properties
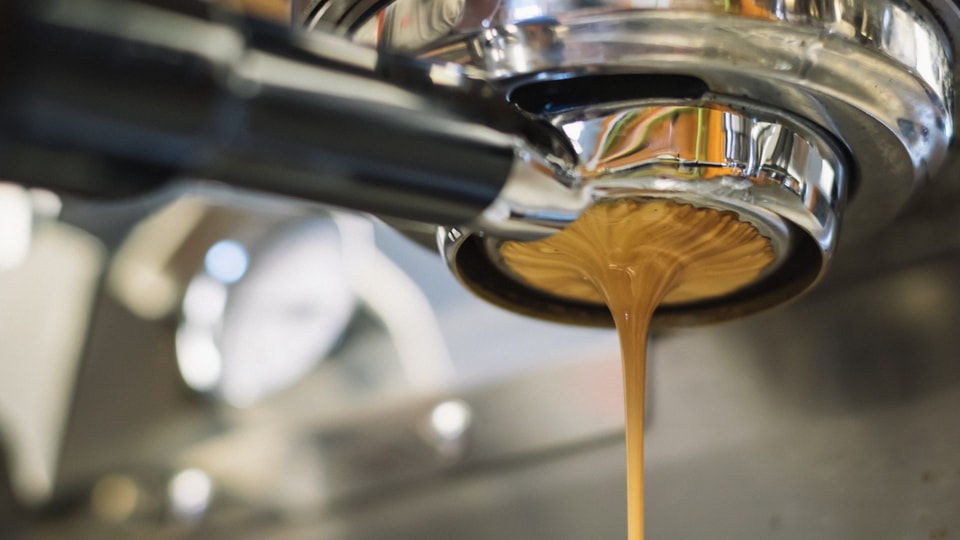
The Problem
How often do you find yourself managing a massive object received from a third-party API or reading data from a JSON file? In JavaScript, objects are everywhere, and many times we need to add, remove, or edit properties of them. And we’d also like to generate a new object so we don’t change the original one.
ECMAScript 5.1 provided us with a wonderful Array.prototype.filter
method to filter arrays and return only lists of values filtered by our condition. But for the same operation on objects, we always have to go for less functional approaches.
The Solution
One option is to filter our object properties. We can create a simple utility to do it.
Now you can easily filter the object properties passing a predicate callback, which return true
or false
in order to filter the object properties.
Last updated: