How to Implement Array Flat and FlatMap Methods in JavaScript
Learn how to use the Array flat and flatMap methods in JavaScript and speed up your development process. Our guide covers implementation details, examples, and more.
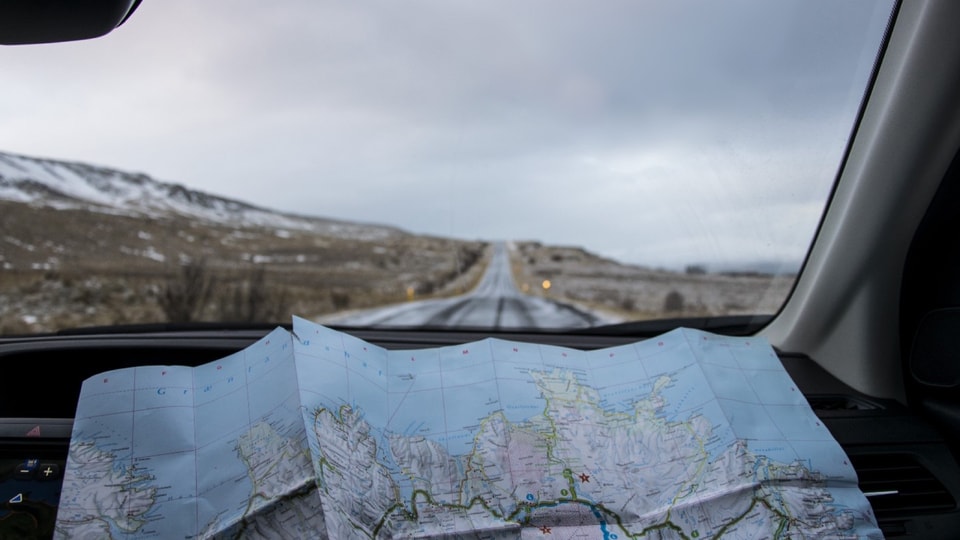
As a programming language, JavaScript is constantly evolving, adding new syntax, features, or abstractions which help developers to easily solve complex problems.
With ES10, we received a couple of new methods for arrays to work on a common operation you’ve probably seen many times in your code: spreading arrays’ elements into a new one.
These two methods are flat
and flatMap
. Let’s talk about what they do and a possible polyfill to simulate their behaviors in old browsers.
flat
The flat
method, part of the array prototype, returns a new array, spreading the content of nested arrays found in the list. It eventually takes an optional depth
argument that represents how many nested levels of our array we want to spread before stopping.
As this method is not fully supported by all the browsers (check the supported list here: Caniuse), let's implement an imperative and performing solution to achieve the same result:
flatMap
The flatMap
method works very similarly to flat
, but it iterates over every element and flats the result of the passed callback function into a new array. We could see it as a combination of the usual map
and flat
methods.
The difference from flat
is that this method only flattens the callback result by one level, and not deeply as flat does.
Last updated: