Building a High-Performance Web Application with Advanced Koa.js Boilerplate
Maximize your web application's performance with this advanced Koa.js boilerplate. Learn how to create a high-performing web application using best practices and advanced techniques to ensure your app is fast and responsive.
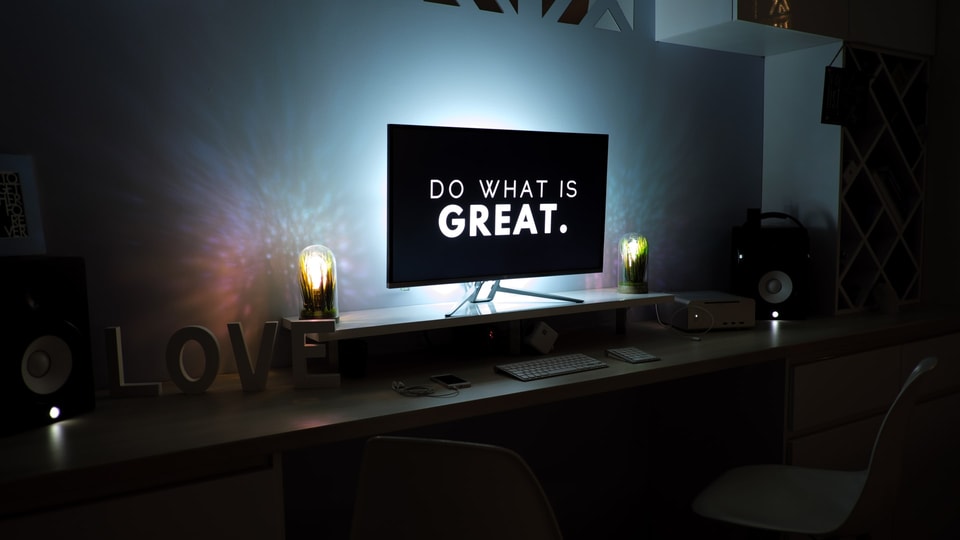
What is Koa???
Over one year and a half ago, I started working with Koa.js at Codeworks, firstly because I love Node.js and everything around its sphere of concern is interesting to me. Furthermore, I was looking for an alternative to Express.js, to replace it with a lighter option. And there I started my path with Koa.js, a framework both powerful and flexible**.**
“Koa is a new web framework designed by the team behind Express, which aims to be a smaller, more expressive, and more robust foundation for web applications and APIs.”
Catching up with the Express little brother was not so difficult: they are both based on the middleware pattern and the concept of asynchronous middleware was not new to me. Moreover, the native ability of Koa of handling them made me very thrilled about this framework.
The problem
After an exciting beginning, I soon realized that something was curious: used to the Express jungle of information available online, every time I was scraping the web for any resource related to Koa, the community was certainly active, but not as much as I expected.
Especially, I struggled to find:
- Middleware packages able to properly solve the corresponding task which Express owns in its core module.
- A best practice error handling tutorial for Koa applications.
- A folder structure to match my idea of scalability.
These are only some of the troubles I encountered starting with Koa, and for anyone obsessed with a structural organization like me, the first problem to solve was how to properly build the skeleton of my application.
After reading hundreds of web articles and having inspiration from developers more skilled than me, I came up with the boilerplate that I currently use to speed up the setup of my Koa applications. So I thought, why shouldn’t I share it with beginners who are probably struggling as I did?
The solution: Create Koa Application
After reviewing my Koa app template (which of course, still needs optimizations), I created a command line tool to allow users to download my boilerplate and initialize a new Koa application on their local machines. Welcome Create Koa Application.
create-koa-application
Having a boilerplate to share is nice, but creating a CLI tool to download it is even better! Two days after I decided to share it, I finished a command line program to download and make the boilerplate ready to use.
It works similarly to the well-known create-react-app, meaning that you can simply write the name of your project after the command and it will generate a folder with the template.
The tool will automatically download the latest version of the template, maintained and updated on a different open-source project (I invite all of you to contribute to making it continuously better!). Therefore, it is not necessary to download or update every time the new version of the CLI.
In case you prefer to not install the tool globally on your local machine, it also works with npx (recommended use!), using the latest version every time you want to create a new project.
The project
The core idea of this boilerplate is to save time setting up the development environment for your app, which will be immediately initialized with:
- eslint configuration to follow clean code conventions and keep it readable.
- prettier configuration to fastly format your code, applying good styling rules.
- jest installation and script to run your test suite.
- husky to easily add your git hooks.
- environment variables compatibility, to make your app follow the best coding practice for configuration files.
In the creation step, create-koa-application copies the template, updates the dependencies (e.g. updates your project name in the packaje.json, etc.), installs them and creates a new git repository if not exist, adding a commit with the new files.
After the installation ends well, your project is ready to use! You can immediately launch your app using one of the scripts already declared in the package.json:
- npm start: Start your Koa application, listening for incoming requests.
- npm run dev: Start your Koa application in development mode. It uses nodemon to refresh your app every time you save changes on your files, perfect to increase the development speed of your project.
- npm run format: Format your code following the prettier rules.
- npm run lint: Lint your code to find style errors.
- npm run check: Run format and lint scripts.
- npm test: Run your test suites.
The folder structure
The boilerplate proposes a model based on a feature-encapsulation pattern (at least, I like to call it so! 😂)which uses a folder for every RESTful API you decide to implement in your app.
The main folders you see on this structure are:
api: it contains an index.js file and a folder for each API. For example, if you want to create an API for users, define your endpoints in the user.routes.js file:
User endpoints
It exports a function that receives the Router module, creates a new sub-router and declares the routes with the related controllers, returning finally the generated instance.
This function will be automatically imported and added to the existing main router by the index.js file in the API folder:
Index file to aggregate all the sub-router
In the end, when required by the server.js file that creates our app, the applyApiMiddleware function applies all the API created and divided by feature. Now you can add a RESTful API for each entity of your project.
config: This folder includes all the configuration files used throughout the whole app. Thanks to joi an object schema validator for JavaScript objects, it reads and validates the configuration from your environment variables, generating a unique config object. This means that you have to only require the
config
folder to have access to the entire configuration object.middleware: In this folder goes the definition of additional middlewares such as an error handler, already implemented, or any other additive middleware you need. For example, here you could add a middleware to validate authenticated users, which may be called auth.middleware.js?! 🤓
utils: The utils folder is the one in charge of containing all the utilities the developer needs to spread through the controller logic or in any part of the application.
Wrapping up
This project comes with the final purpose of helping developers to work on a boilerplate continuously evolving and following the Node.js best practices, integrating advanced patterns.
I hope this resource will be appreciated and I invite all of you with more (or less) experience than me to contribute to this project and make the community around Koa grow faster!
Last updated: