Boost React Performance with 4 Powerful Custom Hooks
Enhance your React app with these 4 custom hooks designed to improve performance, productivity, and the user experience.
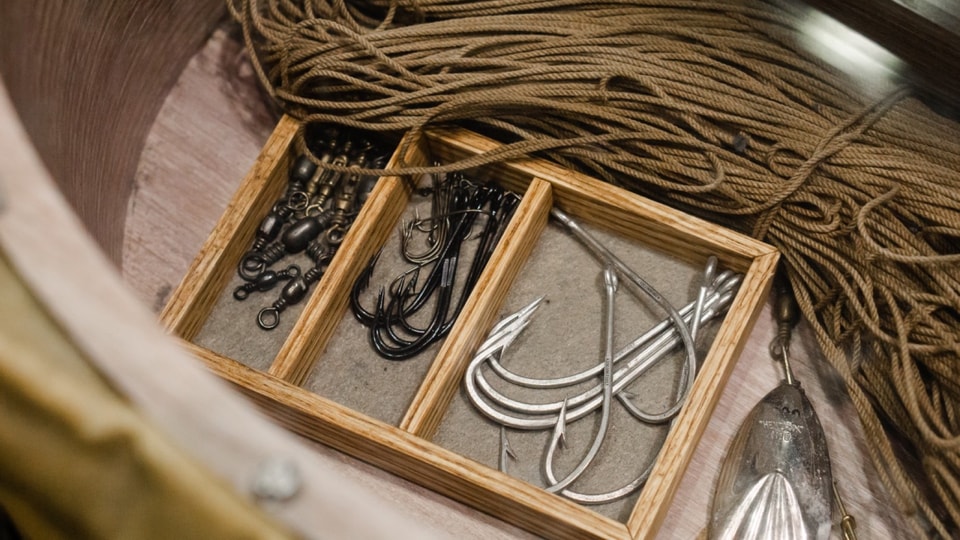
After 9 months since the first stable release, React Hooks have changed the way frontend developers write React components. It’s a normal step in the evolution process of libraries like this, the introduction of new features decides which libraries and frameworks survive over others in the continuous race of frontend development. React was already a highly regarded frontend library, but with the React Hooks introduction, it made a big step.
Why React Hooks?
They help developers to keep control of side effects for our functional components without the need for using a class approach and lifecycle methods such as componentDidMount
, componentDidUpdate
, etc. Furthermore, they allow developers to share logic across components, reducing code duplication and human errors.
React has built-in hooks that provide functionality around the component control process, but the most amazing feature is the ability to combine them to create custom hooks and the ability for hooks to interact with the browser’s API.
Numerous libraries provide custom hooks, and some of those may handle the functionality we’re creating here and in a better way. However, it’s always good to see what happens behind the scene of functions that you already use every day! Let’s write our custom hooks!
usePrefetch
The usePrefetch
hook can help you improve the loading time of the main app page by lazy loading other secondary components that don’t need to render on the first view. The goal is to reduce bundle size and make your application respond quicker.
For our example, let’s assume that we have a page with some information and a button that opens a modal. Before we press the button, the modal shouldn’t render and we don’t need it yet. But if we import it at the beginning of the main component, we will need more time to download its JavaScript code because it will be included in the original bundle. What we want to do is lazy load the modal component to split its code from the main component and prefetch it when the main component has been rendered the first time.
Q.A. Why do we define an **importModal**
function? Because if we write the import inline, it will happen every time a new function is called, and it will run the useEffect
inside the hooks on every render.
useGeo
This hook gets the current position and the updated value whenever the user moves:
Q.A. Why do we define an **isLoad**
variable? Since getting the position is an async operation, it is possible that your component re-renders. So we run the cleanup function before it effectively retrieves the location. With this workaround, we can prevent running the onSuccess
or onFailure
handlers if the component has been unmounted too soon.
useInterval
The useInterval
effect is probably the best-known custom React hook, but here I’ll show its implementation by Dan Abramov since we’ll use it for the next hook:
useTimer
Occasionally developers face the need to implement an efficient way to get a countdown timer, so let’s create a countdown timer starting from a timestamp that represents the amount of time we want to countdown in milliseconds:
Last updated: