How to Use the useViewport Hook in React
Get a step-by-step guide on implementing the useViewport custom hook in React to enhance your app's responsiveness.
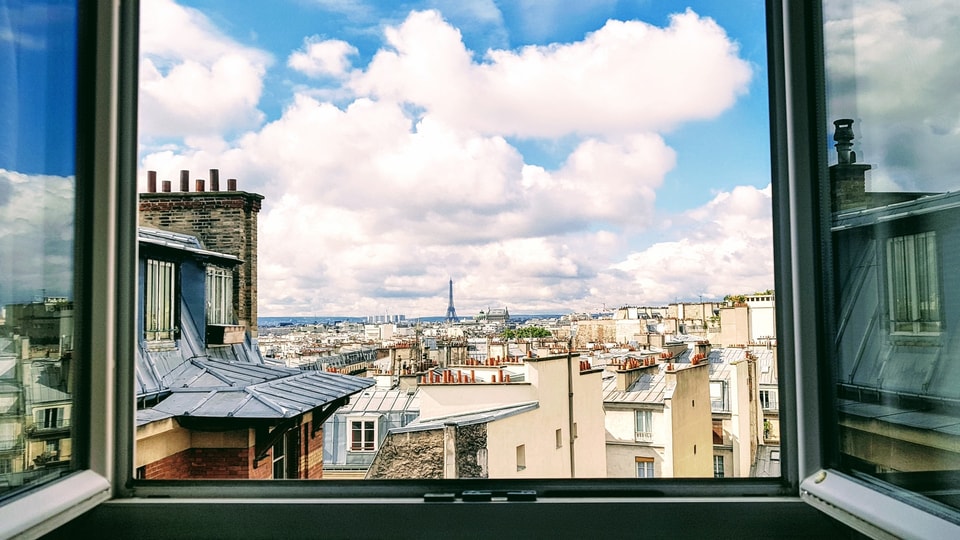
Why do we need info about the viewport?
Working with web applications, we want to provide the best possible experience to our page users. This implies recognising whether it is better to render an element on desktop mode rather than in mobile and vice-versa.
CSS Media queries are great at handling these scenarios, helping to apply the proper styles depending on the screen size.
But sometimes, in order to render the right content on different viewports, we need to manage this logic at a scripting level using JavaScript.
A simple example consists of rendering different texts depending on the screen size:
Fortunately, the React custom hook useMediaQuery
helps on this purpose, providing access to the result of a media query directly from a component written in React.
Then why do I need useViewport?
The useMediaQuery
custom hook is great to retrieve a boolean value for the passed media query, but when using it many times across a codebase, it could make the code a bit unreadable and difficult to understand.
That's why, leveraging the composability of hooks, we can abstract this repeated logic into the useViewport
custom hook.
useViewport technical implementation
What a React developer commonly uses are some boolean flags to know whether the current device has a typical mobile, tablet or desktop viewport size.
This means our custom hook has to return at least these three values, which we'll recall as:
- isMobile
- isTablet
- isDesktop
In this implementation, I'm using the useMediaQuery hook to retrieve whether the device has a mobile or tablet size, and using these two boolean values I can directly classify all the other devices as desktop mode.
Obviously, you can define your media breakpoint as you prefer for your app, those used in the implementation are just examples of is possible to pass a media query to useMediaQuery.
Wrapping up
Now that we have our hook ready, we can finally finish implementing the previous use case we left pending:
Last updated: