5 Signs You’re Becoming a Skilled Programmer
Are you on the right path as a developer? Find out with these 5 signs that show you’re growing into a confident, capable programmer.
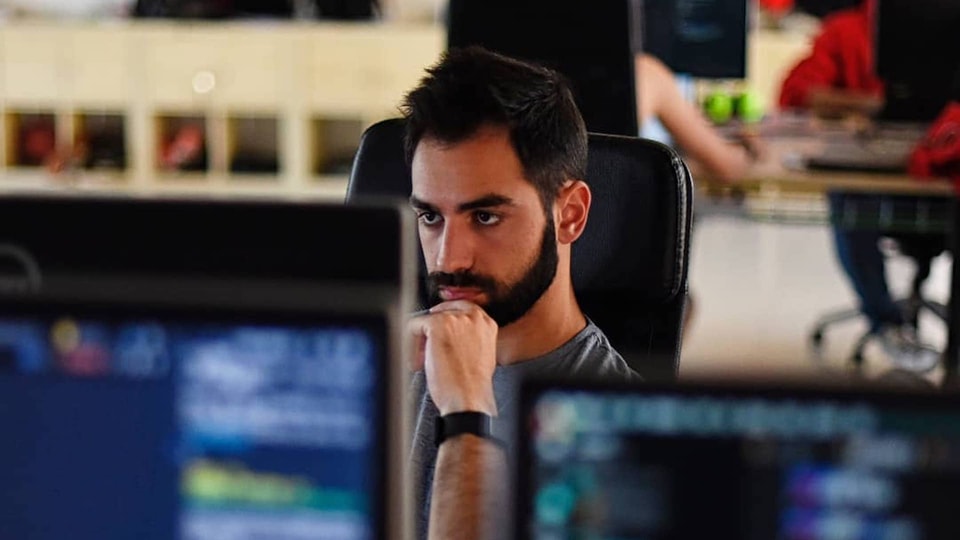
According to SlashData, in 2019 the world reached 26.4 million active software developers.
Whether you’ve been in IT since the beginning of your career or you just started, the question you should ask yourself is the same: Do I really know programming?
The Two Programmers
Though countless shades and differences exist between programmers, I’ve most often met two types in my life: the impostor and the self-confident programmer.
The impostors
Impostors, a category I sometimes include myself in, are those developers who never feel adequate for their role. The constant fear of not being good enough for a job or responsibility seeds doubt that we’re not doing enough to keep up the fast pace of evolving technology, making us feel behind the group.
This often leads to always studying more and more to fill that gap — which, in the process, transforms us into more skilled developers than we even realize.
However, being someone affected by impostor syndrome isn’t something to be ashamed of. Around 70% of all developers have gone through this state of mind at least once in their life, so it’s pretty common, especially at the beginning of one’s career, when what we know is still a drop in the ocean.
The self-confident programmers
On the other hand, some programmers have the exact opposite perspective of their skill level. I’ve passed through a phase of this as well, luckily realizing it before it was too late.
I’m talking about when we become overconfident in our skills and believe we are extreme programmers.
Achieving our first coding successes on simple projects is a really good feeling, of course. It finally shows us that we can do it — that we’re coding. But with great powers shouldn’t come the following aspects:
- Arrogance: Every time we believe our solution is better than someone else’s, we’re taking it one step closer to a closed mindset. In programming, there are tons of solutions to the same problem, and never comparing what we do because we think our solution is the greatest doesn’t help since we limit our chances of seeing the problem from a different perspective.
- Laziness: Just because we cover an important role in our company or we demonstrate a good breadth of knowledge on a specific topic doesn’t mean we’re at the top of our career or that it’s time to take a seat and stop learning. No one will ever know everything, and the moment we stop being curious is the real end of our growth as developers.
Unluckily, I used to be affected by the first (and worst) of these aspects, feeling like a god when I knew nothing about coding. And I still don’t.
But the right people will always help us get better, not only as developers but as human beings as well.
For me, this help came in the form of feedback from my former CTO. I had been working for a bit more than one year at the company, and I was cocky enough to think I was doing good as a programmer and that my level was advanced. I had never been so wrong.
Speaking with him, he helped me realize that my skills as a developer were nothing without the most important one: being able to listen.
When you start listening more to other people’s opinions without rushing in to expose yours, you’ll gain more value in their eyes — and hopefully in your own as well.
This has been a real change for me, and I continue to look at making my future choices hold more of an open view of how to behave as a programmer.
What Do I Know?
To define our programming-knowledge level, we can’t simply ask our grandma if we’re good enough — that doesn’t work since they love us more than anybody else.
To make this distinction, it’s important to define some criteria for establishing our competence level. But before doing that, I’d like to state something I think is important to interiorize:
There are no bad programmers — only less experienced ones.
This is important because it gives a different perspective and reminds each of us of how we were when coding our first lines of code.
So how do we evaluate if we know to code? For this exercise, I’ll refer to “The Four Pillars of a Good Software Developer” described well by Bradley Braithwaite.
Code Quality
When I inspect some of my code, a natural question I ask myself is “Does this snippet look good enough to be understood by myself in one week?”
This makes the code quality aspect much more personal since everyone has a different perspective. However, there’s a list of generic questions we can answer to determine if we effectively respect good standards or if we can improve:
Is my code easily readable?
Code readability should be one of our main concerns. Modern programming languages introduced lots of fancy and short-syntax tricks to allow for less verbose code, creating a lot of one-line coders, who tend to use them to write less.
That’s good for our ego because it makes us proud of the cool feature written in one single line, but when you go to check the same code after six weeks, you’ll have loved it if you had used a more descriptive syntax of what that snippet was supposed to do.
What we tend to forget is that while it may not be difficult for a computer to understand our code, for humans it is. We should write our code so people can understand it. For a computer, those lines will get converted to 0s and 1s, so it doesn’t matter if you used a cool syntax or something more descriptive.
“Code is for humans.”
Is my code consistent?
Being consistent when writing code is as important as writing clean code.
You can follow your favorite code style — it doesn’t matter. What is essential for keeping your code well-written and easy to read is to stick to that coding style so that others reading your code base can easily navigate it.
There are plenty of good guidelines that can help us to discover what our coding style should be, especially some well-written books — which I recommended in “4 Books Everyone in Tech Should Read and Why.” They’ve been essential to me in building my coding practices.
Code Correctness
Now that we got an idea of how we should write our code to keep it clean and maintainable, there’s a little detail we should take care of: Your code should work as expected.
What I mean is we should write code that not only matches the requirements we have for it but also takes care of corner cases and error cases. Just to go straight to the point, check the following snippet, where we want to write a function that sums two numbers:
Do you see what I mean? This function is receiving two numbers and returns their sum, but it only covers the best scenario. In case we pass something different from numbers as parameters, it’ll behave differently from the required functionality.
This simple example gives us some questions to answer:
- Do I cover edge cases and different behaviors in my code?
- Do I handle errors in case they occur in my code?
- Do I test properly my code functionalities?
- Does this code depend on external dependencies I should consider?
We can keep these questions as a checklist our code should respect when creating new functionalities (or refactoring them). Of course, it’s not always possible to do everything perfectly, but always aiming to solidify our code into its role is a good way to become a great developer!
“Perfection is not attainable, but if we chase perfection we can catch excellence.”
Code Performance
After seeing that our code looks good, is maintainable, and satisfies all the correctness requirements, we can start going deep down into the rabbit hole of software programming and talk about performance.
I care about how performant my code is, and this usually is the biggest mistake I keep repeating: Writing fast and reliable code is important, but overthinking your code and including premature optimizations can destroy your productivity and slow down functionality.
We can’t neglect the need to write our implementation so users can enjoy a smooth experience with our software. However, we should do it step by step.
The 5 commandments of performant code
There are some simple rules I try to follow (not always successfully) that are a good starting point:
- Make it work. Then refactor. Then deep optimize.
- Don’t trade code quality for code performance (if the app isn’t strictly performance-sensitive).
- If the code has external dependencies, check how they work before optimizing.
- Measure performance when integrating new code to immediately detect possible deoptimizations.
- If it’s an architectural decision, deeply think about the right data structure to use.
Following these suggestions will make you slower in the beginning, but repeating them in cycles and making mistakes, in the long term, will improve the way you work, allowing you to automatically follow better practices and reduce errors.
Code Productivity
With practice, all developers in the long term get better. They learn to apply solid programming principles and know how to write professional code. Something that may distinguish the excellent developer from simply the good developer is how productive they are in producing their qualitative code.
Here again, we could ask ourselves some questions:
- Do I know my coding rhythm? Getting an idea of what distracts us, how often we need a break, or what makes us delay our tasks is the first step in preventing that situation where you find yourself looking at your screen with no idea of how to implement something. Get to know yourself and your habits to be able to fully concentrate and stay productive.
- Do I automate my tasks? Programmers are people who program, so who’s better than us to give instructions to our devices on how to do tasks we repeat many times?
For example, I wrote a script to automate a release process at our company, which save us much more time than we could have expected on every iteration. - Do I know my tools? Thanks to recent progress in software development, we have plenty of amazing tools, such as IDEs, task managers, terminals, and much more. Using them at 100% of their potential can boost our productivity so much — soon we’ll forget how we ever used to be so slow at moving the mouse across the screen just to close windows. Whenever you start using a new tool, inspect all the possibilities it has, and learn how to integrate them into your workflow.
The Fifth Pillar
In the beginning, I said to refer to the four pillars to answer our question about how much we know about programming, but that was a lie. There’s a last pillar that I consider the most important — the one that characterizes the best developers.
It’s about communication. The greatest developers in the world are those able to communicate and explain easily how their code works or makes concrete an abstraction.
Teachers, conference speakers, writers … all of them not only prove to be great programmers but also be able to make others great, giving pills of wisdom in a way that would be more difficult to learn alone.
“If you can’t explain it simply, you don’t understand it well enough.”
Wrapping Up
This has been a long reflection I started some time ago that I wanted to share with you. I hope you’ll reap the benefits of asking more questions of yourself — and answering them, as I did.
Last updated: